NavigationDrawer + ToolBar + Fragment
1. activity_main.xml에서 drawerlayout 사용 NavigationView 추가 후
2. header 레이아웃 파일 추가 - header.xml
3. 메뉴파일 추가 -> drawer_menu.xml ,
4. toolbar_menu.xml
5. 스타일에서 액션바 사용안하도록 지정
6. string.xml 에서 open, close 추가
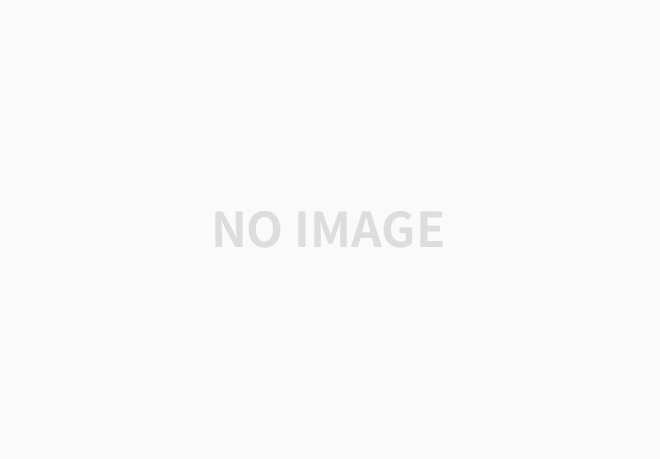
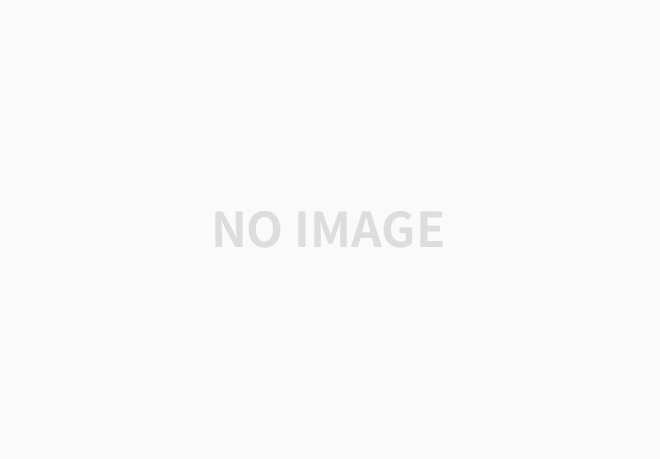
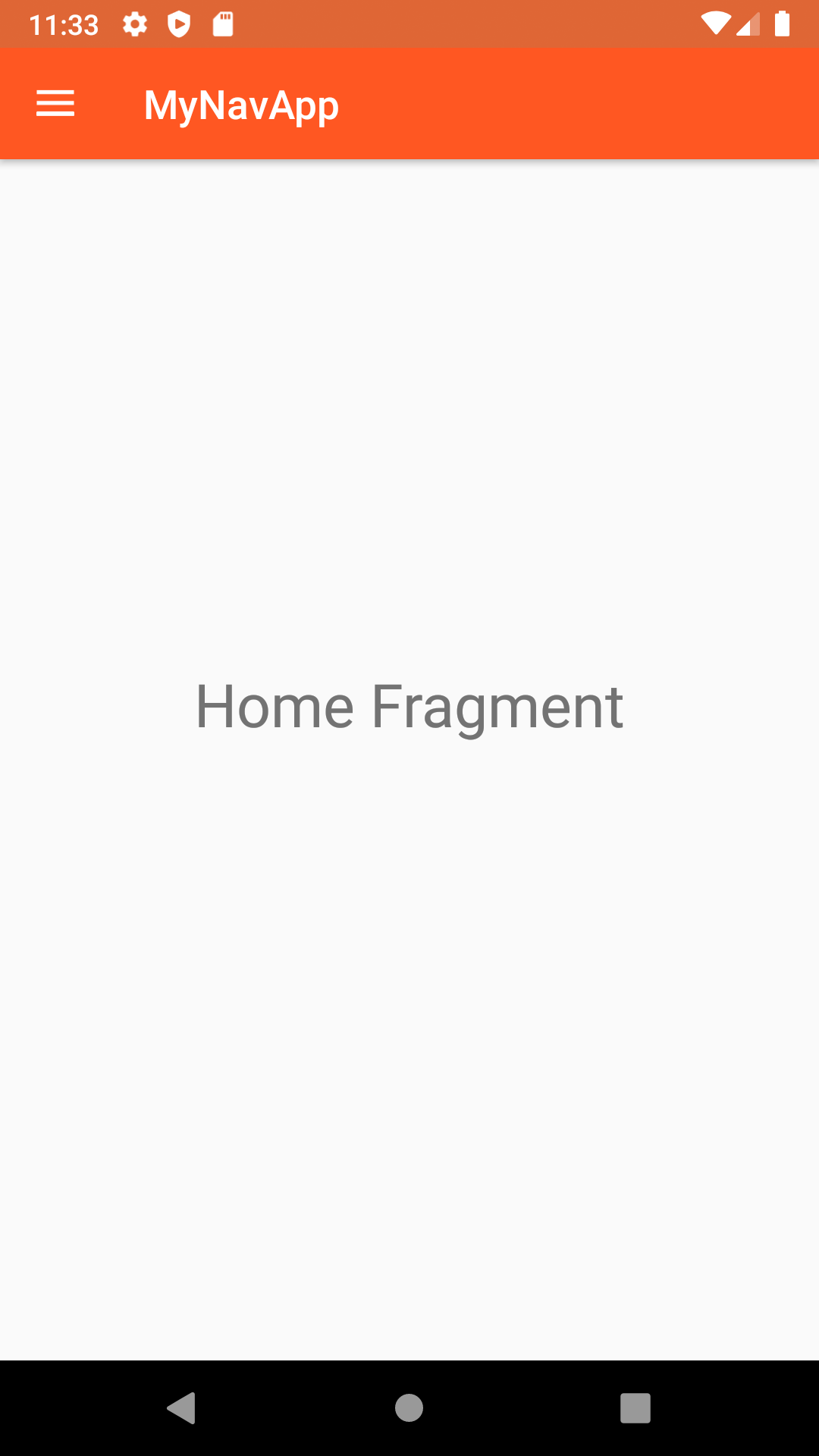
1. gradle
//add dependency
implementation 'com.google.android.material:material:1.0.0'
implementation 'androidx.navigation:navigation-fragment:2.0.0'
implementation 'androidx.navigation:navigation-ui:2.0.0'
2. Fragment 생성 with layout xml file
HomeFragment, SchoolFragment, WorkFragment, SettingFragment, TimeLineFragment, LogoutFragment
package com.nurisoft.navigationdrawertoolbarfragment;
import android.os.Bundle;
import androidx.fragment.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
/**
* A simple {@link Fragment} subclass.
*/
public class HomeFragment extends Fragment {
public HomeFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_home, container, false);
}
}
3. app_bar_main layout xml file
actionbar를 대체 할 상단 AppBarLayout과 본문 부분 fragment가 위치할 FrameLayout를 배치함
<?xml version="1.0" encoding="utf-8"?>
<androidx.coordinatorlayout.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto">
<com.google.android.material.appbar.AppBarLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:theme="@style/AppTheme.AppBarOverlay"
>
<androidx.appcompat.widget.Toolbar
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:id="@+id/toolBar"
android:background="@color/colorPrimary"
app:popupTheme="@style/AppTheme.PopupOverlay"
/>
</com.google.android.material.appbar.AppBarLayout>
<!--for navigation drawer i will use fragments so for fragment i
will use framelayout-->
<FrameLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/frame_layout"
>
</FrameLayout>
</androidx.coordinatorlayout.widget.CoordinatorLayout>
values > styles.xml
<resources>
<!-- Base application theme. -->
<style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar">
<!-- Customize your theme here. -->
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
</style>
<style name="AppTheme.NoActionBar">
<item name="windowActionBar">false</item>
<item name="windowNoTitle">true</item>
</style>
<style name="AppTheme.AppBarOverlay" parent="ThemeOverlay.AppCompat.Dark.ActionBar" />
<style name="AppTheme.PopupOverlay" parent="ThemeOverlay.AppCompat.Light" />
</resources>
values > strings.xml
<resources>
<string name="app_name">MyNavApp</string>
<string name="open">open</string>
<string name="close">close</string>
<!-- TODO: Remove or change this placeholder text -->
<string name="hello_blank_fragment">Hello blank fragment</string>
</resources>
values > colors.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="colorPrimary">#FF5722</color>
<color name="colorPrimaryDark">#DF6635</color>
<color name="colorAccent">#D81B60</color>
<color name="whiteColor">#ffffff</color>
</resources>
AndroidManifest.xml에 NoActionBar 설정
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.nurisoft.navigationdrawertoolbarfragment">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity"
android:theme="@style/AppTheme.NoActionBar">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
4. Navigation Drawer의 Head 부분 레이아웃 파일 생성 (header.xml)
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:gravity="bottom"
android:background="@drawable/background"
android:layout_width="match_parent"
android:layout_height="176dp"
android:padding="16dp"
>
<!--for backgroud i have an image-->
<ImageView
android:layout_width="75dp"
android:layout_height="75dp"
android:paddingTop="8dp"
android:src="@mipmap/ic_launcher_round"
/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Android WorldClub"
android:textColor="@color/whiteColor"
android:textSize="20sp"
android:textStyle="bold"
android:layout_marginTop="10dp"
/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="androidworldclub@gmail.com"
android:textColor="@color/whiteColor"
android:textSize="18sp"
/>
</LinearLayout>
5. Navigation Drawer 의 메뉴부분 (drawer_menu.xml) 생성
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
tools:showIn="navigation_view">
<!--now add some icons-->
<group android:checkableBehavior="single">
<item
android:id="@+id/home"
android:title="Home"
android:icon="@drawable/ic_action_home"
/>
<item
android:id="@+id/work"
android:title="Work"
android:icon="@drawable/ic_action_work"
/>
<item
android:id="@+id/school"
android:title="School"
android:icon="@drawable/ic_action_shool"
/>
<item
android:id="@+id/timeline"
android:title="Time Line"
android:icon="@drawable/ic_action_timeline"
/>
</group>
<item android:title="Controls">
<menu>
<item
android:id="@+id/setting"
android:title="Setting"
android:icon="@drawable/ic_action_setting"
/>
<item
android:id="@+id/logout"
android:title="Logout"
android:icon="@drawable/ic_action_logout"
/>
</menu>
</item>
</menu>
6. 메인레이아웃 (activity_main.xml)
<?xml version="1.0" encoding="utf-8"?>
<androidx.drawerlayout.widget.DrawerLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/drawerLayout"
android:fitsSystemWindows="true"
tools:context=".MainActivity">
<include
layout="@layout/app_bar_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
/>
<com.google.android.material.navigation.NavigationView
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:id="@+id/nav_view"
android:background="@color/whiteColor"
app:menu="@menu/drawer_menu"
app:headerLayout="@layout/header"
android:fitsSystemWindows="true"
android:layout_gravity="start"
>
</com.google.android.material.navigation.NavigationView>
</androidx.drawerlayout.widget.DrawerLayout>
7. MainActivity.java
package com.nurisoft.navigationdrawertoolbarfragment;
import androidx.annotation.NonNull;
import androidx.appcompat.app.ActionBarDrawerToggle;
import androidx.appcompat.app.AppCompatActivity;
import androidx.appcompat.widget.Toolbar;
import androidx.core.view.GravityCompat;
import androidx.drawerlayout.widget.DrawerLayout;
import androidx.fragment.app.FragmentTransaction;
import android.os.Bundle;
import android.view.MenuItem;
import com.google.android.material.navigation.NavigationView;
public class MainActivity extends AppCompatActivity implements NavigationView.OnNavigationItemSelectedListener {
DrawerLayout mDrawerLayout;
ActionBarDrawerToggle mDrawerToggle;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Toolbar 셋팅
Toolbar toolbar = findViewById(R.id.toolBar);
setSupportActionBar(toolbar);
// DrawerLayout 셋팅
mDrawerLayout = findViewById(R.id.drawerLayout);
mDrawerToggle = new ActionBarDrawerToggle(this, mDrawerLayout, toolbar, R.string.open, R.string.close);
mDrawerLayout.addDrawerListener(mDrawerToggle);
mDrawerToggle.syncState();
// 메뉴틀릭시 이벤트 셋팅
NavigationView navigationView = findViewById(R.id.nav_view);
navigationView.setNavigationItemSelectedListener(this);
// 프래그먼트
HomeFragment fragment = new HomeFragment();
FragmentTransaction fragmentTransaction = getSupportFragmentManager().beginTransaction();
fragmentTransaction.replace(R.id.frame_layout, fragment, "Home");
fragmentTransaction.commit();
// so now implement onNavigationItemselected
}
@Override
public boolean onNavigationItemSelected(@NonNull MenuItem menuItem) {
int id = menuItem.getItemId();
if (id == R.id.home) {
HomeFragment fragment = new HomeFragment();
FragmentTransaction fragmentTransaction = getSupportFragmentManager().beginTransaction();
fragmentTransaction.replace(R.id.frame_layout, fragment, "Home");
fragmentTransaction.commit();
}
else if (id == R.id.work) {
WorkFragment fragment = new WorkFragment();
FragmentTransaction fragmentTransaction = getSupportFragmentManager().beginTransaction();
fragmentTransaction.replace(R.id.frame_layout, fragment, "Work");
fragmentTransaction.commit();
}
else if (id == R.id.school) {
SchoolFragment fragment = new SchoolFragment();
FragmentTransaction fragmentTransaction = getSupportFragmentManager().beginTransaction();
fragmentTransaction.replace(R.id.frame_layout, fragment, "School");
fragmentTransaction.commit();
}
else if (id == R.id.timeline) {
TimeLineFragment fragment = new TimeLineFragment();
FragmentTransaction fragmentTransaction = getSupportFragmentManager().beginTransaction();
fragmentTransaction.replace(R.id.frame_layout, fragment, "TimeLine");
fragmentTransaction.commit();
}
else if (id == R.id.setting) {
SettingFragment fragment = new SettingFragment();
FragmentTransaction fragmentTransaction = getSupportFragmentManager().beginTransaction();
fragmentTransaction.replace(R.id.frame_layout, fragment, "Setting");
fragmentTransaction.commit();
}
else if (id == R.id.logout) {
LogoutFragment fragment = new LogoutFragment();
FragmentTransaction fragmentTransaction = getSupportFragmentManager().beginTransaction();
fragmentTransaction.replace(R.id.frame_layout, fragment, "Logout");
fragmentTransaction.commit();
}
mDrawerLayout.closeDrawer(GravityCompat.START);
return true;
}
@Override
public void onBackPressed() {
if (mDrawerLayout.isDrawerOpen(GravityCompat.START)) {
mDrawerLayout.closeDrawer(GravityCompat.START);
}
else {
super.onBackPressed();
}
}
}
'안드로이드 앱 개발' 카테고리의 다른 글
Toolbar + FloatingActionMenu (0) | 2020.02.12 |
---|---|
RecyclerView + ViewPager + TabLayout (0) | 2020.02.11 |
RecyclerView + Realm + Swip Delete + FloatingActionButton (0) | 2020.02.11 |
RecyclerView + SQLite + Swip Delete + FloatingActionButton (0) | 2020.02.11 |
RecyclerView + SampleData + Filterable SearchView (1) | 2020.02.11 |